Dynamic Web Development with JavaScript and Node.js: Building Interactive and Scalable Applications
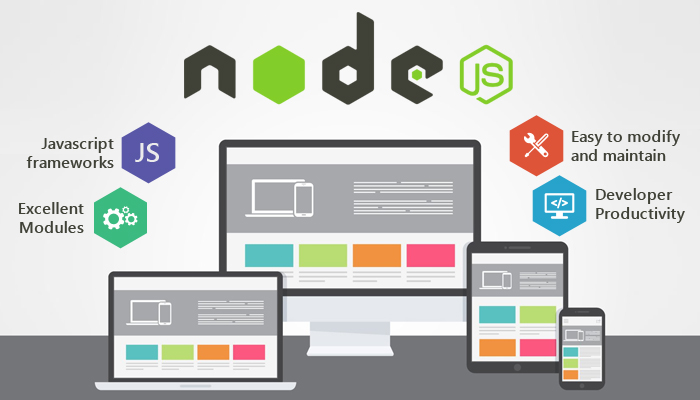
In the realm of web development, creating interactive and scalable applications is a perpetual pursuit. JavaScript, with its versatility and ubiquity, stands at the forefront of this endeavor. Coupled with the server-side runtime environment Node.js, developers can unleash the full potential of dynamic web development. This extensive guide navigates the landscape of dynamic web development, unraveling the intricacies of JavaScript and Node.js to empower developers in building applications that are both interactive and scalable.
I. The Power of JavaScript in Web Development
A. Client-Side Scripting
- JavaScript Fundamentals:
- Description: Grasp the fundamentals of JavaScript, from variables and data types to control flow and functions. Lay the foundation for client-side scripting, enabling the creation of dynamic content within web browsers.
- DOM Manipulation:
- Description: Explore Document Object Model (DOM) manipulation using JavaScript. Learn how to dynamically alter HTML and CSS, providing a seamless and responsive user experience.
B. Asynchronous JavaScript
- Callbacks and Promises:
- Description: Dive into asynchronous programming paradigms with callbacks and promises. Understand how these concepts facilitate non-blocking operations, essential for handling tasks like fetching data and handling user interactions.
- Async/Await:
- Description: Embrace modern asynchronous programming using async/await syntax. Streamline code readability and maintainability while handling asynchronous operations with ease.
II. Introduction to Node.js
A. Server-Side JavaScript
- Node.js Fundamentals:
- Description: Introduce Node.js as a server-side runtime environment for executing JavaScript. Understand the event-driven, non-blocking architecture that sets Node.js apart in the server-side realm.
- NPM (Node Package Manager):
- Description: Navigate the Node Package Manager (NPM) ecosystem. Learn to leverage NPM to install, manage, and share JavaScript packages, enhancing the efficiency of web development projects.
III. Building Interactive Web Applications
A. Server-Side Rendering (SSR)
- Express.js Framework:
- Description: Explore the Express.js framework for building robust web applications. Uncover the simplicity and flexibility it offers in setting up routes, handling requests, and implementing middleware.
- Dynamic Templating with EJS:
- Description: Implement dynamic templating using Embedded JavaScript (EJS). Understand how EJS enables the server to dynamically generate HTML, responding to user inputs and changing application states.
B. Real-Time Communication
- WebSockets and Socket.IO:
- Description: Dive into real-time communication between clients and servers using WebSockets. Explore Socket.IO as a powerful library facilitating bidirectional, event-driven communication in real time.
- Building a Chat Application:
- Description: Apply real-time communication concepts by building a chat application. Witness how WebSockets and Socket.IO enable instant message delivery, creating a dynamic and engaging user experience.
IV. Scalability with Node.js
A. Microservices Architecture
- Introduction to Microservices:
- Description: Explore the concept of microservices architecture. Understand how breaking down applications into smaller, independently deployable services enhances scalability and maintainability.
- Building Microservices with Node.js:
- Description: Dive into building microservices using Node.js. Learn the principles of service-oriented architecture, inter-service communication, and deploying microservices for a scalable application ecosystem.
B. Load Balancing and Clustering
- Load Balancing Strategies:
- Description: Grasp load balancing strategies to distribute incoming traffic across multiple Node.js instances. Explore approaches like round-robin, least connections, and IP hash for optimal resource utilization.
- Node.js Clustering:
- Description: Implement Node.js clustering to take advantage of multi-core systems. Understand how clustering enables parallel processing, improving application performance and responsiveness.
V. Data Management in Node.js
A. Database Integration
- MongoDB and Mongoose:
- Description: Integrate MongoDB, a NoSQL database, with Node.js using Mongoose. Explore the flexibility of document-oriented databases and leverage Mongoose for seamless data modeling and interaction.
- SQL Databases with Sequelize:
- Description: Connect Node.js applications to SQL databases using Sequelize. Understand how an Object-Relational Mapping (ORM) library simplifies database interactions, providing a structured approach to data management.
B. RESTful API Development
- Designing RESTful APIs:
- Description: Delve into the principles of Representational State Transfer (REST) and design RESTful APIs. Learn best practices for creating scalable, resource-oriented APIs that facilitate seamless communication between client and server.
- Express.js for API Development:
- Description: Utilize Express.js for building robust APIs. Implement routes, middleware, and request handling to create a RESTful API that supports CRUD operations and adheres to industry standards.
VI. Testing and Debugging Node.js Applications
A. Unit Testing and Debugging Tools
- Mocha and Chai:
- Description: Embrace the Mocha testing framework and Chai assertion library for unit testing Node.js applications. Understand the importance of writing testable code and ensuring the reliability of your applications.
- Debugging with VS Code:
- Description: Utilize Visual Studio Code (VS Code) as a powerful tool for debugging Node.js applications. Explore breakpoints, watches, and other debugging features to identify and resolve issues effectively.
VII. Security Best Practices
A. Securing Node.js Applications
- OWASP Top Ten:
- Description: Familiarize yourself with the OWASP Top Ten, a list of the most critical security risks for web applications. Implement security measures to mitigate vulnerabilities and protect Node.js applications from common threats.
- Middleware for Security:
- Description: Implement middleware for enhancing the security of Node.js applications. Explore concepts such as authentication, authorization, and encryption to fortify your applications against potential security breaches.
VIII. Deployment and Performance Optimization
A. Deploying Node.js Applications
- Containerization with Docker:
- Description: Containerize Node.js applications using Docker. Understand how containerization streamlines deployment, ensures consistency across environments, and facilitates the scaling of applications.
- Continuous Integration and Deployment (CI/CD):
- Description: Implement CI/CD pipelines for automated testing, integration, and deployment of Node.js applications. Explore tools like Jenkins or GitHub Actions to establish efficient and reliable deployment workflows.
B. Performance Optimization
- Caching Strategies:
- Description: Optimize the performance of Node.js applications with caching strategies. Explore techniques like in-memory caching, CDN caching, and database query caching to reduce response times and enhance scalability.
- Monitoring and Profiling:
- Description: Implement monitoring and profiling tools to gain insights into the performance of Node.js applications. Explore solutions like New Relic, AppDynamics, or built-in Node.js tools to identify bottlenecks and optimize code for efficiency.
IX. Future Trends in JavaScript and Node.js
A. Serverless Architectures
- Introduction to Serverless:
- Description: Explore the concept of serverless architectures. Understand how platforms like AWS Lambda and Azure Functions enable developers to build scalable applications without managing traditional server infrastructure.
- Functions as a Service (FaaS):
- Description: Dive into Functions as a Service (FaaS) with Node.js. Discover how serverless functions allow developers to focus on writing code while leveraging the scalability and cost-effectiveness of serverless platforms.
X. Conclusion
Dynamic web development with JavaScript and Node.js unfolds as a journey marked by continuous exploration, innovation, and adaptation. Armed with the knowledge embedded in this comprehensive guide, developers embark on a quest to build applications that not only respond dynamically to user interactions but also scale gracefully to meet growing demands. The synergy between JavaScript’s client-side capabilities and Node.js’s server-side prowess forms the backbone of a technology landscape that thrives on responsiveness, interactivity, and scalability. As the evolution of web development persists, JavaScript and Node.js remain stalwart companions, empowering developers to shape the future of dynamic and scalable applications. Embrace the dynamic nature of the web, harness the power of JavaScript and Node.js, and propel your applications into the realms of interactivity and scalability. The journey begins here, with a commitment to the art and craft of dynamic web development.