Mastering Machine Learning with Python and Scikit-learn
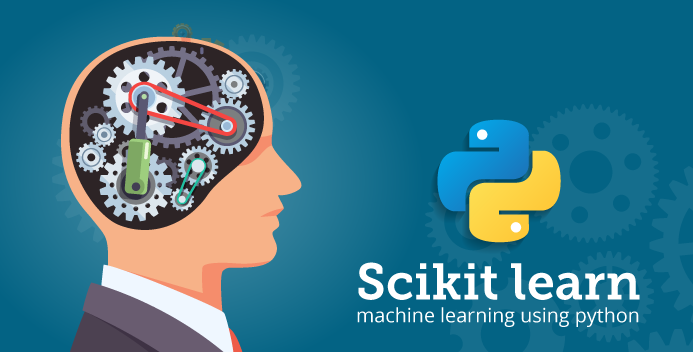
Introduction to Scikit-learn
Scikit-learn is a powerful and versatile Python library for machine learning. It provides a consistent interface to a wide range of algorithms for classification, regression, clustering, and more. This comprehensive guide will delve into the core concepts and practical applications of scikit-learn.
Essential Libraries
Before diving into machine learning, ensure you have the necessary libraries installed:
pip install numpy scipy matplotlib scikit-learn
- NumPy: Provides support for large, multi-dimensional arrays and matrices.
- SciPy: Offers scientific computing routines.
- Matplotlib: Used for data visualization.
- Scikit-learn: The machine learning library.
Data Preparation
Machine learning models rely on quality data. Here’s a breakdown of essential data preprocessing steps:
Loading Data
- CSV:
pandas.read_csv()
- Excel:
pandas.read_excel()
- Databases:
pandas.read_sql()
Data Exploration
- Descriptive statistics:
describe()
- Visualization:
matplotlib
andseaborn
- Correlation analysis:
pandas.corr()
Data Cleaning
- Handling missing values:
fillna()
,dropna()
- Outlier detection:
z-score
, IQR - Feature scaling:
StandardScaler
,MinMaxScaler
- Encoding categorical features:
LabelEncoder
,OneHotEncoder
Feature Engineering
- Creating new features: Derived attributes from existing data.
- Feature selection: Identifying relevant features.
Model Selection and Training
Scikit-learn offers a wide range of algorithms for different machine learning tasks:
Supervised Learning
- Classification:
- Logistic Regression
- Support Vector Machines (SVM)
- Naive Bayes
- Decision Trees
- Random Forest
- K-Nearest Neighbors (KNN)
- Regression:
- Linear Regression
- Ridge Regression
- Lasso Regression
- Decision Trees
- Random Forest
Unsupervised Learning
- Clustering:
- K-Means
- Hierarchical Clustering
- Dimensionality Reduction:
- Principal Component Analysis (PCA)
- t-SNE
Model Training and Evaluation
- Splitting data:
train_test_split
- Model fitting:
fit()
method - Model evaluation:
accuracy_score
,mean_squared_error
,confusion_matrix
- Cross-validation:
cross_val_score
Model Optimization
- Hyperparameter tuning:
GridSearchCV
,RandomizedSearchCV
- Regularization: Prevent overfitting
- Ensemble methods: Combine multiple models
Model Deployment
- Serialization:
pickle
,joblib
- Model serving: Flask, Django, FastAPI
- Cloud platforms: AWS, GCP, Azure
Advanced Topics
- Pipeline: Streamline the machine learning workflow.
- Feature importance: Understand feature contributions.
- Imbalanced datasets: Handle class imbalance.
- Model interpretation: Explainable AI techniques.
- Deep learning integration: Combine scikit-learn with deep learning frameworks.
Case Studies
To solidify your understanding, consider applying scikit-learn to real-world problems:
- Customer churn prediction: Classify customers likely to churn.
- Fraud detection: Build a model to identify fraudulent transactions.
- Recommendation systems: Develop a product recommendation engine.
- Image classification: Create models to classify images.
- Natural language processing: Analyze text data.
Conclusion
Scikit-learn is a powerful tool for building machine learning models in Python. By mastering its core concepts and techniques, you can effectively tackle a wide range of data-driven problems. Continuous learning and experimentation are key to becoming proficient in machine learning.