How to Use PyQt for GUI Development
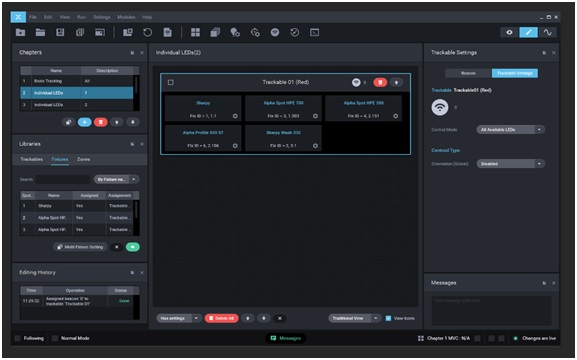
Introduction to PyQt
PyQt is a Python binding for the Qt application framework, enabling the creation of cross-platform GUI applications. It offers a rich set of tools for building complex user interfaces.
Installation
To install PyQt, use pip:
pip install PyQt5
Replace PyQt5
with PyQt6
for the latest version.
Basic Structure of a PyQt Application
A typical PyQt application follows these steps:
- Import necessary modules:Python
import sys from PyQt5.QtWidgets import QApplication, QWidget, QLabel
- Create a QApplication instance:Python
app = QApplication(sys.argv)
- Create widgets:Python
window = QWidget() label = QLabel("Hello, World!")
- Set up layout (optional):Python
# ...
- Show the window:Python
window.show()
- Start the event loop:Python
sys.exit(app.exec_())
Core Widgets
PyQt offers a wide range of widgets for building user interfaces:
- QLabel: Displays text or images.
- QPushButton: Creates clickable buttons.
- QLineEdit: Provides a single-line text input field.
- QTextEdit: Provides a multi-line text editing area.
- QComboBox: Creates dropdown lists.
- QListWidget: Displays a list of items.
- QTableWidget: Displays data in a tabular format.
- QProgressBar: Displays progress bars.
- QCheckBox: Creates checkboxes.
- QRadioButton: Creates radio buttons.
Layouts
To arrange widgets within a window, PyQt provides several layout managers:
- QHBoxLayout: Arranges widgets horizontally.
- QVBoxLayout: Arranges widgets vertically.
- QGridLayout: Arranges widgets in a grid layout.
- BoxLayout: A generic layout that can be used for both horizontal and vertical layouts.
Signals and Slots
PyQt uses a signal-slot mechanism for communication between objects. A signal is emitted by an object when something happens (e.g., button clicked), and a slot is a function that is called in response to a signal.
button.clicked.connect(my_function)
Event Handling
PyQt allows you to handle various events, such as mouse clicks, key presses, and window resizing.
def on_click():
# Handle button click
button.clicked.connect(on_click)
Custom Widgets
For more complex UI components, you can create custom widgets by subclassing existing widgets or creating widgets from scratch.
Dialogs
PyQt provides several standard dialogs:
- QMessageBox: Displays message boxes with various buttons.
- QFileDialog: Opens file dialogs for selecting files or directories.
- QColorDialog: Opens a color selection dialog.
- QFontDialog: Opens a font selection dialog.
Designing Complex GUIs
For complex GUIs, consider using Qt Designer, a visual tool for creating UI forms. You can then convert the generated UI file to Python code using the pyuic
tool.
Additional Topics
- Styling with Stylesheets: Customize the appearance of your application using CSS-like stylesheets.
- Model-View Architecture: Separate data from presentation for better maintainability.
- Threading: Use threads for background tasks to prevent UI freezing.
- Database Integration: Connect your GUI application to databases.
- Deployment: Package your application for distribution.
Conclusion
PyQt is a powerful and versatile tool for building desktop applications. By mastering its core concepts, widgets, and layout management, you can create professional-looking and user-friendly GUIs.