How to Use Map, Filter, and Reduce in Python
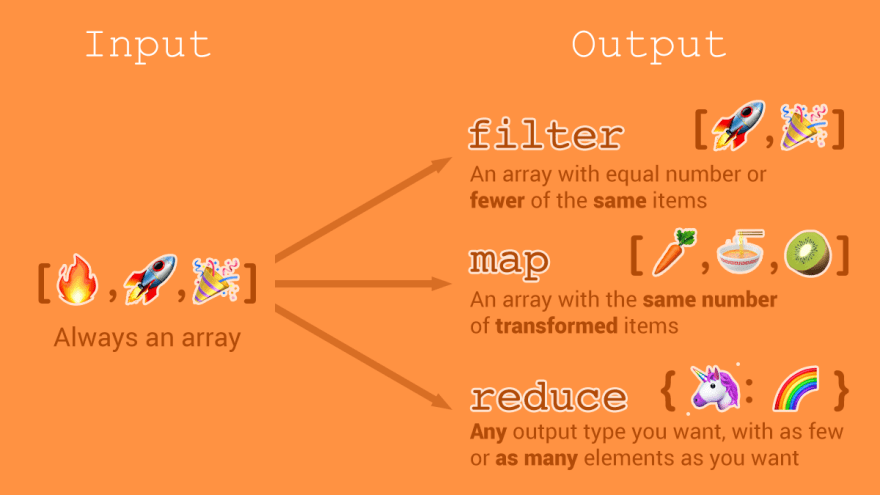
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. In Python, the map
, filter
, and reduce
functions are key tools that support functional programming. These functions allow you to write clean, concise, and efficient code. This article will provide an in-depth explanation of how to use these functions, including practical examples and scenarios where they are particularly useful.
Understanding map
, filter
, and reduce
Before diving into detailed examples, it’s important to understand what each of these functions does:
map
: Applies a given function to all items in an input list (or any iterable) and returns an iterator that produces the results.filter
: Constructs an iterator from elements of an iterable for which a function returns true.reduce
: Applies a rolling computation to sequential pairs of values in a list (or any iterable), reducing the iterable to a single cumulative value.
Using map
The map
function is used to apply a function to all items in an input list. The general syntax is:
map(function, iterable, ...)
Example: Squaring Numbers
Suppose you have a list of numbers and you want to create a new list with the squares of these numbers. You can achieve this using the map
function.
numbers = [1, 2, 3, 4, 5]# Define a function that squares a number
def square(num):
return num ** 2
# Use map to apply the function to each item in the list
squared_numbers = list(map(square, numbers))
print(squared_numbers)
Alternatively, you can use a lambda function for simplicity:
squared_numbers = list(map(lambda num: num ** 2, numbers))
print(squared_numbers)
Example: Converting Temperatures
If you have a list of temperatures in Celsius and want to convert them to Fahrenheit, you can use the map
function.
celsius = [0, 10, 20, 30, 40]# Define a function that converts Celsius to Fahrenheit
def to_fahrenheit(c):
return (c * 9/5) + 32
# Use map to apply the function to each item in the list
fahrenheit = list(map(to_fahrenheit, celsius))
print(fahrenheit)
Using filter
The filter
function constructs an iterator from elements of an iterable for which a function returns true. The general syntax is:
filter(function, iterable)
Example: Filtering Even Numbers
Suppose you have a list of numbers and you want to create a new list containing only the even numbers. You can achieve this using the filter
function.
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]# Define a function that checks if a number is even
def is_even(num):
return num % 2 == 0
# Use filter to apply the function to each item in the list
even_numbers = list(filter(is_even, numbers))
print(even_numbers)
Again, you can use a lambda function for simplicity:
even_numbers = list(filter(lambda num: num % 2 == 0, numbers))
print(even_numbers)
Example: Filtering Positive Numbers
If you have a list of numbers and want to filter out only the positive numbers, you can use the filter
function.
numbers = [-10, -5, 0, 5, 10]# Define a function that checks if a number is positive
def is_positive(num):
return num > 0
# Use filter to apply the function to each item in the list
positive_numbers = list(filter(is_positive, numbers))
print(positive_numbers)
Using reduce
The reduce
function from the functools
module applies a rolling computation to sequential pairs of values in a list, reducing the iterable to a single cumulative value. The general syntax is:
from functools import reduce
reduce(function, iterable, [initializer])
Example: Summing Numbers
Suppose you have a list of numbers and you want to calculate their sum. You can achieve this using the reduce
function.
from functools import reducenumbers = [1, 2, 3, 4, 5]
# Define a function that adds two numbers
def add(x, y):
return x + y
# Use reduce to apply the function cumulatively to the items in the list
total = reduce(add, numbers)
print(total)
Using a lambda function:
total = reduce(lambda x, y: x + y, numbers)
print(total)
Example: Finding the Maximum Value
If you have a list of numbers and want to find the maximum value, you can use the reduce
function.
numbers = [1, 5, 3, 9, 2]# Define a function that returns the maximum of two numbers
def max(x, y):
return x if x > y else y
# Use reduce to apply the function cumulatively to the items in the list
max_value = reduce(max, numbers)
print(max_value)
Using a lambda function:
max_value = reduce(lambda x, y: x if x > y else y, numbers)
print(max_value)
Combining map
, filter
, and reduce
You can combine map
, filter
, and reduce
to perform complex data manipulations in a concise and readable manner. Let’s look at a few examples.
Example: Summing the Squares of Even Numbers
Suppose you have a list of numbers and you want to find the sum of the squares of the even numbers. You can achieve this by combining map
, filter
, and reduce
.
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]# Filter even numbers, map to their squares, and reduce to their sum
sum_of_squares = reduce(
lambda x, y: x + y,
map(
lambda num: num ** 2,
filter(lambda num: num % 2 == 0, numbers)
)
)
print(sum_of_squares)
Example: Finding the Product of Positive Numbers
If you have a list of numbers and want to find the product of all positive numbers, you can combine map
, filter
, and reduce
.
numbers = [-10, -5, 0, 5, 10]# Filter positive numbers and reduce to their product
product_of_positives = reduce(
lambda x, y: x * y,
filter(lambda num: num > 0, numbers)
)
print(product_of_positives)
Practical Scenarios
Data Processing and Transformation
When working with large datasets, you often need to transform data from one format to another. map
is particularly useful for this purpose.
Example: Converting Strings to Integers
string_numbers = ["1", "2", "3", "4", "5"]# Use map to convert each string to an integer
integer_numbers = list(map(int, string_numbers))
print(integer_numbers)
Filtering Data
Filtering is a common task when processing data, especially when you need to clean or refine datasets.
Example: Filtering Invalid Data
data = [15, -3, 22, None, 45, '', 8]# Use filter to remove None and empty string values
clean_data = list(filter(lambda x: x is not None and x != '', data))
print(clean_data)
Aggregating Data
Aggregating data, such as calculating sums, averages, or other cumulative statistics, is often necessary in data analysis.
Example: Calculating the Average
numbers = [1, 2, 3, 4, 5]# Calculate the sum and count using reduce, then compute the average
total = reduce(lambda x, y: x + y, numbers)
count = len(numbers)
average = total / count
print(average)
Conclusion
The map
, filter
, and reduce
functions are powerful tools for functional programming in Python. They allow you to write clean, concise, and efficient code for a variety of data processing tasks. By understanding how to use these functions and combining them effectively, you can handle complex data manipulations with ease.
In this article, we’ve explored the syntax and use cases for each of these functions, provided practical examples, and demonstrated how to combine them for more advanced operations. Whether you’re transforming, filtering, or aggregating data, map
, filter
, and reduce
offer elegant solutions that enhance the readability and maintainability of your code.
As you continue to develop your Python skills, incorporating these functional programming concepts into your toolkit will help you write more robust and versatile programs.