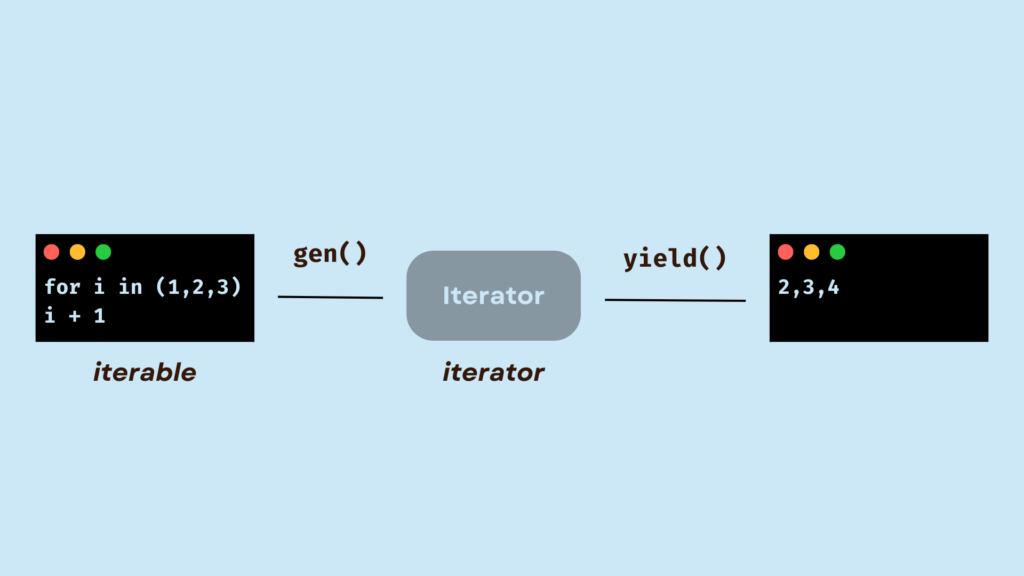
Generators are a special class of functions in Python that simplify the task of writing iterators. They allow you to iterate over data without the need to store the entire dataset in memory at once, making them an essential tool for handling large data streams efficiently. This article will cover the basics of generators, how they differ from regular functions and iterators, and explore various use cases and advanced techniques.
What is a Generator?
A generator is a function that returns an iterator object which we can iterate over (one value at a time). Generators use the yield
keyword instead of return
to produce a series of values lazily. When a generator function is called, it does not execute immediately but returns a generator object. Execution of the function’s code is deferred until next()
is called on the generator object.
Differences Between Generators and Regular Functions
- Memory Efficiency: Generators do not store the entire dataset in memory. Instead, they generate values on the fly, which is particularly useful for large datasets.
- State Retention: Generators automatically maintain their state between iterations. They pause their execution and retain their local variables until the next value is requested.
- Single Use: Unlike lists or tuples, generators can be iterated over only once. Once all values have been generated, they cannot be restarted.
Creating Generators
Generator Functions
Generator functions use the yield
keyword to produce a sequence of values. Here’s a basic example:
def count_up_to(max):
count = 1
while count <= max:
yield count
count += 1
counter = count_up_to(5)
for num in counter:
print(num)
Output:
1
2
3
4
5
In this example, the count_up_to
function generates numbers from 1 to max
.
Generator Expressions
Generator expressions provide a concise way to create generators using a syntax similar to list comprehensions. They use parentheses instead of square brackets:
squares = (n**2 for n in range(10))
for square in squares:
print(square)
Output:
0
1
4
9
16
25
36
49
64
81
Use Cases for Generators
Reading Large Files
Generators are particularly useful for reading large files where loading the entire file into memory is not feasible:
def read_large_file(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line.strip()
for line in read_large_file('large_file.txt'):
print(line)
This approach reads one line at a time, making it memory efficient.
Generating Infinite Sequences
Generators are ideal for generating infinite sequences, such as Fibonacci numbers:
def fibonacci():
a, b = 0, 1
while True:
yield a
a, b = b, a + b
fib = fibonacci()
for _ in range(10):
print(next(fib))
Output:
0
1
1
2
3
5
8
13
21
34
Pipeline Processing
Generators can be used to create data processing pipelines. Here’s an example of a simple pipeline that processes a list of numbers:
def generate_numbers():
for i in range(1, 11):
yield idef square_numbers(numbers):
for number in numbers:
yield number**2
def filter_even(numbers):
for number in numbers:
if number % 2 == 0:
yield number
numbers = generate_numbers()
squares = square_numbers(numbers)
even_squares = filter_even(squares)
for num in even_squares:
print(num)
Output:
4
16
36
64
100
Advanced Generator Techniques
Using yield
in Loops
Generators can use yield
in loops to produce multiple values:
def countdown(n):
while n > 0:
yield n
n -= 1
for num in countdown(5):
print(num)
Output:
5
4
3
2
1
Generator Chaining
Generators can be chained together to form complex data processing pipelines:
def chain_generators(gen1, gen2):
yield from gen1
yield from gen2gen1 = (x for x in range(5))
gen2 = (x for x in range(5, 10))
for value in chain_generators(gen1, gen2):
print(value)
Output:
0
1
2
3
4
5
6
7
8
9
Bidirectional Communication
Generators can receive data from the caller using the send()
method:
def echo():
while True:
received = yield
print(f'Received: {received}')
generator = echo()
next(generator) # Prime the generator
generator.send('Hello')
generator.send('World')
Output:
Received: Hello
Received: World
Performance Considerations
Generators are more memory efficient than lists, especially when dealing with large datasets or infinite sequences. However, the lazy evaluation of generators means that they can be slower than lists in some scenarios where all elements are needed immediately.
Debugging Generators
Debugging generators can be tricky due to their lazy nature. Here are some tips:
- Use Logging: Add logging statements within the generator to track its execution flow.
- Prime Generators: Ensure generators are properly primed using
next()
orsend(None)
. - Convert to List: Temporarily convert the generator to a list to inspect its output.
Common Pitfalls
- Single Use: Generators can be iterated over only once. If you need to iterate multiple times, convert the generator to a list.
- Uninitialized State: Generators must be primed before using
send()
. Callnext()
orsend(None)
to start the generator. - Generator Expression Scope: Variables used in generator expressions can lead to unexpected behavior if they are modified elsewhere.
Conclusion
Generators are a powerful feature in Python, offering memory efficiency and the ability to handle large or infinite data streams. By understanding the basics and advanced techniques, you can leverage generators to write more efficient and readable code.
Practice Problems
- Prime Numbers Generator: Create a generator that produces an infinite sequence of prime numbers.python
def is_prime(n):
if n < 2:
return False
for i in range(2, int(n**0.5) + 1):
if n % i == 0:
return False
return Truedef prime_numbers():
n = 2
while True:
if is_prime(n):
yield n
n += 1primes = prime_numbers()
for _ in range(10):
print(next(primes))
- File Chunk Reader: Write a generator that reads a file in fixed-size chunks.python
def read_file_in_chunks(file_path, chunk_size=1024):
with open(file_path, 'rb') as file:
while chunk := file.read(chunk_size):
yield chunkfor chunk in read_file_in_chunks('large_file.txt'):
print(chunk)
- Cyclic Generator: Create a generator that cycles through a given list indefinitely.python
def cyclic_generator(iterable):
while True:
for item in iterable:
yield itemcolors = ['red', 'green', 'blue']
cycle = cyclic_generator(colors)
for _ in range(10):
print(next(cycle))
By practicing these problems and incorporating generators into your coding routine, you will become proficient in writing more efficient and scalable Python code. Happy coding!
4o