How to Create a GUI with Tkinter
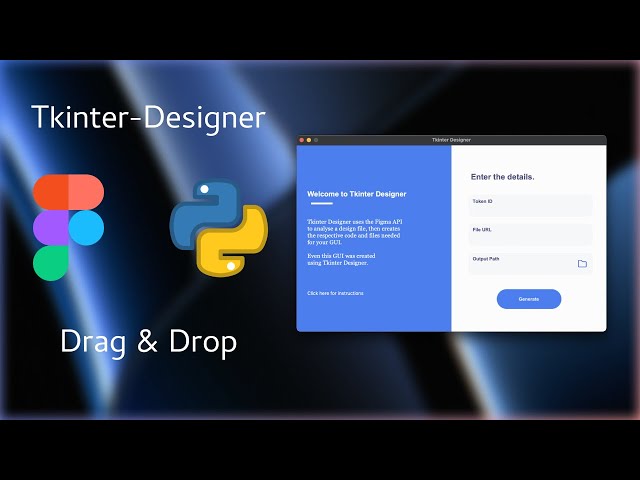
Creating a Graphical User Interface (GUI) is a crucial part of software development that allows users to interact with applications in an intuitive way. Tkinter, the standard GUI library for Python, offers a simple and effective way to design and implement GUIs. This comprehensive guide will cover everything you need to know about creating a GUI with Tkinter, including its fundamentals, widgets, layout management, and advanced features.
Table of Contents
- Introduction to Tkinter
- Setting Up Your Environment
- Basic Tkinter Window
- Tkinter Widgets
- Layout Management
- Event Handling and Callbacks
- Advanced Widgets
- Styling and Theming
- Integration with Other Libraries
- Debugging and Optimization
- Best Practices
- Conclusion
1. Introduction to Tkinter
What is Tkinter?
Tkinter is Python’s standard library for creating graphical user interfaces. It is a wrapper around the Tcl/Tk GUI toolkit and provides a simple way to create windows, dialogs, buttons, labels, and other common GUI elements.
Advantages of Tkinter
- Standard Library: Tkinter comes bundled with Python, so there’s no need for external installations.
- Simplicity: Tkinter is easy to learn and use, making it ideal for beginners.
- Cross-Platform: Tkinter applications run on Windows, macOS, and Linux.
- Rich Widget Set: Provides a range of widgets to create functional and interactive UIs.
2. Setting Up Your Environment
Installing Python
Ensure that you have Python installed on your system. Tkinter is included with Python by default. You can download Python from the official Python website.
Verifying Tkinter Installation
To check if Tkinter is installed, you can use the following Python command:
import tkinter
print(tkinter.TkVersion)
If Tkinter is installed, this will print the version number of Tkinter.
3. Basic Tkinter Window
Creating a Simple Window
To create a basic Tkinter window, follow these steps:
- Import the Tkinter module.
- Create an instance of the
Tk
class. - Set the window title and dimensions.
- Start the Tkinter event loop.
import tkinter as tk# Create the main window
root = tk.Tk()
root.title("My First Tkinter App")
root.geometry("400x300") # Set window size
# Run the Tkinter event loop
root.mainloop()
Explanation
tk.Tk()
creates the main application window.title()
sets the window’s title.geometry()
defines the window’s size.mainloop()
starts the event handling loop, which keeps the window open.
4. Tkinter Widgets
Labels
Labels are used to display text or images.
label = tk.Label(root, text="Hello, Tkinter!")
label.pack()
Buttons
Buttons are interactive elements that trigger actions.
def on_button_click():
print("Button clicked!")button = tk.Button(root, text="Click Me", command=on_button_click)
button.pack()
Entry Widgets
Entry widgets are used for single-line text input.
entry = tk.Entry(root)
entry.pack()
Text Widgets
Text widgets allow for multi-line text input and display.
text = tk.Text(root, height=10, width=40)
text.pack()
Checkboxes
Checkboxes are used to make binary choices.
checkbox = tk.Checkbutton(root, text="Check me")
checkbox.pack()
Radio Buttons
Radio buttons are used for selecting one option from a set.
radio_var = tk.StringVar()
radio1 = tk.Radiobutton(root, text="Option 1", variable=radio_var, value="1")
radio2 = tk.Radiobutton(root, text="Option 2", variable=radio_var, value="2")
radio1.pack()
radio2.pack()
Listbox
A listbox displays a list of items from which the user can select.
listbox = tk.Listbox(root)
listbox.insert(1, "Item 1")
listbox.insert(2, "Item 2")
listbox.pack()
5. Layout Management
Pack Geometry Manager
The pack()
method organizes widgets in blocks before placing them in the parent widget.
frame = tk.Frame(root)
frame.pack(side=tk.LEFT, padx=10, pady=10)
label = tk.Label(frame, text="Packed Label")
label.pack()
Grid Geometry Manager
The grid()
method arranges widgets in a grid of rows and columns.
label1 = tk.Label(root, text="Name:")
label1.grid(row=0, column=0)
entry1 = tk.Entry(root)
entry1.grid(row=0, column=1)
Place Geometry Manager
The place()
method places widgets at an absolute position.
button = tk.Button(root, text="Place Me")
button.place(x=50, y=50)
6. Event Handling and Callbacks
Binding Events
Tkinter allows you to bind events to callback functions.
def on_key_press(event):
print("Key pressed:", event.keysym)root.bind("<KeyPress>", on_key_press)
Button Click Event
You can attach a function to a button click using the command
parameter.
def on_button_click():
print("Button clicked!")button = tk.Button(root, text="Click Me", command=on_button_click)
button.pack()
Handling User Input
Use callback functions to process user input from widgets like Entry or Text.
def submit_form():
user_input = entry.get()
print("User input:", user_input)submit_button = tk.Button(root, text="Submit", command=submit_form)
submit_button.pack()
7. Advanced Widgets
Menus
Menus provide a way to group related commands.
def open_file():
print("Open File")menu = tk.Menu(root)
root.config(menu=menu)
file_menu = tk.Menu(menu)
menu.add_cascade(label="File", menu=file_menu)
file_menu.add_command(label="Open", command=open_file)
Dialogs
Tkinter provides dialogs for user interactions, like message boxes and file dialogs.
from tkinter import messageboxdef show_message():
messagebox.showinfo("Message", "Hello, World!")
button = tk.Button(root, text="Show Message", command=show_message)
button.pack()
Canvas
The Canvas widget allows for custom drawing.
canvas = tk.Canvas(root, width=200, height=200)
canvas.pack()
canvas.create_line(0, 0, 200, 200)
canvas.create_rectangle(50, 50, 150, 150, fill="blue")
Frames
Frames are used to group and organize widgets.
frame = tk.Frame(root, bg="lightgrey")
frame.pack(padx=10, pady=10)label = tk.Label(frame, text="Inside Frame")
label.pack()
8. Styling and Theming
Changing Widget Colors
You can customize the appearance of widgets using options like bg
for background color and fg
for text color.
button = tk.Button(root, text="Styled Button", bg="blue", fg="white")
button.pack()
Fonts
Change the font and size of text in widgets.
label = tk.Label(root, text="Styled Text", font=("Helvetica", 16))
label.pack()
Themes
Tkinter supports themes through the ttk
module, which provides themed widgets.
from tkinter import ttkstyle = ttk.Style()
style.configure('TButton', background='green', foreground='white')
button = ttk.Button(root, text="Themed Button")
button.pack()
9. Integration with Other Libraries
Integrating with Databases
Tkinter can be integrated with databases like SQLite to create data-driven applications.
import sqlite3conn = sqlite3.connect('example.db')
cursor = conn.cursor()
cursor.execute('CREATE TABLE IF NOT EXISTS users (id INTEGER PRIMARY KEY, name TEXT)')
conn.commit()
conn.close()
Plotting with Matplotlib
Tkinter can be used with Matplotlib to create interactive plots.
import matplotlib.pyplot as plt
from matplotlib.backends.backend_tkagg import FigureCanvasTkAggfig, ax = plt.subplots()
ax.plot([1, 2, 3], [4, 5, 6])
canvas = FigureCanvasTkAgg(fig, master=root)
canvas.get_tk_widget().pack()
10. Debugging and Optimization
Debugging Tips
- Use Print Statements: Add print statements to check the flow of execution.
- Check for Exceptions: Look for and handle exceptions that may occur during execution.
- Use Debugging Tools: Utilize Python’s built-in debugger (
pdb
) or IDE debugging tools.
Optimizing Performance
- Reduce Redraws: Minimize unnecessary updates to the GUI.
- Use Efficient Layouts: Choose appropriate layout managers to avoid performance issues.
- Manage Resources: Release resources such as file handles and database connections when not in use.
11. Best Practices
Maintainability
- Organize Code: Use functions and classes to structure your code.
- Document Your Code: Add comments and documentation for clarity.
User Experience
- Design Intuitively: Create a user-friendly and intuitive interface.
- Test Thoroughly: Test your application across different platforms and scenarios.
Security
- Validate Input: Ensure user input is validated and sanitized.
- Handle Errors Gracefully: Provide meaningful error messages and handle exceptions.
12. Conclusion
Tkinter provides a powerful yet accessible toolkit for creating graphical user interfaces in Python. Its simplicity and ease of use make it an excellent choice for both beginners and experienced developers. By understanding the fundamentals, mastering widgets and layout management, and implementing advanced features, you can create sophisticated and user-friendly applications.
Whether you’re building a simple tool or a complex application, Tkinter’s flexibility and integration capabilities allow you to bring your ideas to life with an intuitive and interactive interface. With this comprehensive guide, you are well-equipped to start creating your own Tkinter-based GUIs and exploring the possibilities of Python’s standard GUI library.