Mastering Recursion in Scheme: Techniques, Examples, and Best Practices
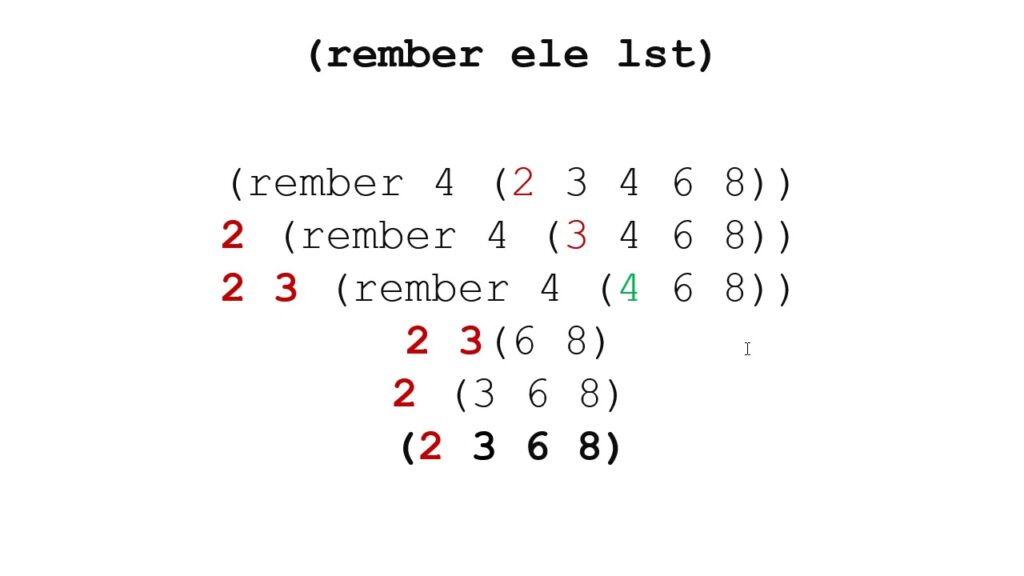
Introduction: Recursion is a fundamental concept in computer science and functional programming, allowing functions to call themselves to solve problems by breaking them down into smaller, simpler subproblems. Scheme, a dialect of Lisp, is well-suited for recursive programming due to its functional nature and support for tail call optimization. By mastering recursion in Scheme, you can write elegant, concise, and efficient code to solve a wide range of problems. In this comprehensive guide, we’ll explore everything you need to know about implementing recursion in Scheme, from basic concepts to advanced techniques and best practices.
- Understanding Recursion Basics: Recursion is a programming technique where a function calls itself with smaller input values until it reaches a base case, where the recursion stops. In Scheme, recursion is a natural and powerful way to solve problems by breaking them down into smaller, more manageable subproblems.
- Writing Recursive Functions in Scheme: In Scheme, recursive functions typically follow a simple pattern: define a base case that specifies the termination condition, and define a recursive case that calls the function with smaller input values. Here’s an example of a simple recursive function in Scheme to calculate the factorial of a non-negative integer:
(define (factorial n)
(if (= n 0)
1
(* n (factorial (- n 1)))))
In this example, the base case is when n equals 0, and the recursive case calculates the factorial of n by multiplying n with the factorial of (n – 1).
- Handling Tail Recursion: Tail recursion is a special form of recursion where the recursive call is the last operation performed by the function. Scheme implementations typically optimize tail-recursive calls to avoid stack overflow errors and improve performance. To ensure tail recursion, use an accumulator parameter to accumulate the result as the function recurses. Here’s an example of a tail-recursive factorial function in Scheme:
(define (factorial n)
(define (factorial-iter n acc)
(if (= n 0)
acc
(factorial-iter (- n 1) (* acc n))))
(factorial-iter n 1))
In this example, the accumulator parameter acc is used to accumulate the factorial result as the function recurses, ensuring tail recursion.
- Applying Recursion to List Processing: Recursion is commonly used in Scheme for processing lists, such as traversing, searching, filtering, and mapping elements. Here’s an example of a recursive function in Scheme to calculate the sum of all elements in a list:
(define (sum lst)
(if (null? lst)
0
(+ (car lst) (sum (cdr lst)))))
In this example, the function recursively sums the car (first element) of the list with the sum of the cdr (rest of the list) until reaching the base case of an empty list.
- Using Mutual Recursion: Mutual recursion is a technique where two or more functions call each other in a cyclic manner to solve a problem. Scheme supports mutual recursion, allowing functions to collaborate and solve complex problems by dividing them into smaller subproblems. Here’s an example of mutual recursion in Scheme to check if a number is even or odd:
(define (even? n)
(if (= n 0)
#t
(odd? (- n 1))))(define (odd? n)
(if (= n 0)
#f
(even? (- n 1))))
In this example, the even? function calls the odd? function, and vice versa, until reaching the base case of n equaling 0.
- Best Practices for Recursion in Scheme: To write efficient, elegant, and maintainable recursive code in Scheme, consider following these best practices:
- Define clear base cases: Ensure that your recursive functions have well-defined base cases that specify when the recursion should stop.
- Use tail recursion: Whenever possible, use tail recursion to optimize recursive calls and avoid stack overflow errors.
- Keep track of state: If necessary, use accumulator parameters to maintain state or accumulate results during tail-recursive calls.
- Test your code: Write comprehensive unit tests to verify the correctness and performance of your recursive functions, covering different input cases and edge conditions.
- Understand termination conditions: Make sure you understand the termination conditions of your recursive functions to avoid infinite loops or excessive resource consumption.
- Document your code: Use comments and documentation to explain the purpose, behavior, and termination conditions of your recursive functions for future reference and maintenance.
- Conclusion: In conclusion, mastering recursion in Scheme is essential for solving a wide range of problems in functional programming, list processing, and algorithm design. By understanding recursion basics, writing recursive functions, handling tail recursion, applying recursion to list processing, using mutual recursion, and following best practices, you can write elegant, efficient, and maintainable code in Scheme. So dive into recursion, practice these techniques, and unlock the full power of Scheme for solving complex problems in a functional and elegant manner.