Mastering Web API Development in Flask: Techniques, Best Practices, and Advanced Features
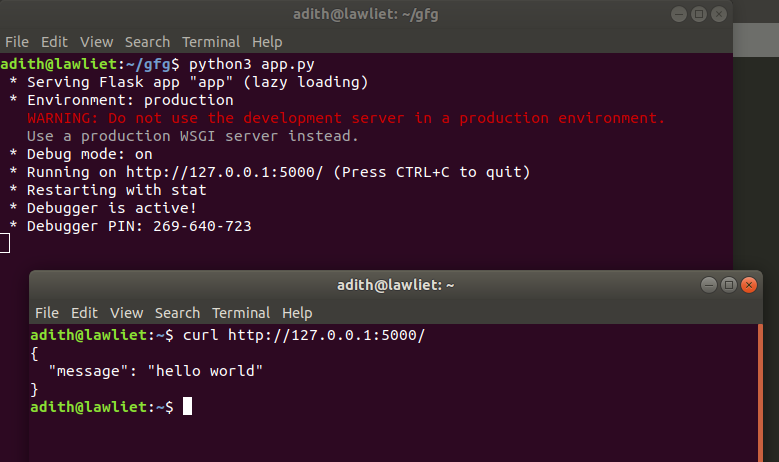
Introduction: Web APIs (Application Programming Interfaces) play a crucial role in modern web development, enabling communication and data exchange between different systems, applications, and devices. Flask, a lightweight and flexible web framework for Python, provides powerful tools and features for building web APIs quickly and efficiently. By mastering web API development in Flask, you can create robust, scalable, and secure APIs to serve data and interact with clients over the web. In this comprehensive guide, we’ll explore everything you need to know about creating web APIs in Flask, from basic concepts to advanced techniques and best practices.
- Understanding Web APIs and RESTful Architecture: Before diving into Flask web API development, it’s essential to understand the concepts of web APIs and RESTful architecture:
- Web API: A web API is an interface that allows different software systems to communicate and interact over the web by sending and receiving HTTP requests and responses. APIs define endpoints, request methods, data formats, and authentication mechanisms for accessing and manipulating resources.
- RESTful Architecture: REST (Representational State Transfer) is a software architectural style for designing networked applications based on a set of principles, such as stateless communication, resource-based URLs, and uniform interfaces. RESTful APIs use HTTP methods (GET, POST, PUT, DELETE) to perform CRUD (Create, Read, Update, Delete) operations on resources represented by URIs (Uniform Resource Identifiers).
- Setting Up Flask for Web API Development: To get started with Flask web API development, you need to install Flask and set up a basic project structure. Here’s a simple example of creating a Flask application for building a web API:
from flask import Flaskapp = Flask(__name__)
def hello():
return 'Hello, World!'
if __name__ == '__main__':
app.run(debug=True)
In this example, we define a Flask route ‘/api/hello’ that returns a simple message when accessed.
- Handling HTTP Methods and Requests: Flask allows you to define routes for different HTTP methods (GET, POST, PUT, DELETE) to handle various types of requests. Here’s an example of handling POST requests to create a new resource in a Flask web API:
from flask import Flask, request, jsonifyapp = Flask(__name__)
def create_user():
data = request.json
# Process request data and create new user
return jsonify({'message': 'User created successfully'})
if __name__ == '__main__':
app.run(debug=True)
In this example, we define a route ‘/api/users’ that accepts POST requests to create new user resources.
- Returning JSON Responses: JSON (JavaScript Object Notation) is a lightweight data interchange format commonly used in web APIs for transmitting structured data between clients and servers. Flask provides built-in support for returning JSON responses using the jsonify function. Here’s an example of returning JSON responses in a Flask web API:
from flask import Flask, jsonifyapp = Flask(__name__)
def get_data():
data = {'name': 'John', 'age': 30, 'city': 'New York'}
return jsonify(data)
if __name__ == '__main__':
app.run(debug=True)
In this example, we define a route ‘/api/data’ that returns a JSON object containing sample data.
- URL Parameters and Query Strings: Flask allows you to capture URL parameters and query strings from incoming requests to provide dynamic and customizable behavior in your web APIs. Here’s an example of handling URL parameters and query strings in a Flask web API:
from flask import Flask, request, jsonifyapp = Flask(__name__)
def get_user(user_id):
# Retrieve user data based on user ID
return jsonify({'user_id': user_id})
def search_users():
query = request.args.get('query')
# Perform search based on query string
return jsonify({'query': query})
if __name__ == '__main__':
app.run(debug=True)
In this example, we define routes ‘/api/user/<user_id>’ and ‘/api/search’ to handle requests with URL parameters and query strings, respectively.
- Error Handling and Exception Handling: Error handling is an essential aspect of web API development to handle unexpected errors and provide meaningful responses to clients. Flask allows you to define custom error handlers and exception handlers to handle various types of errors gracefully. Here’s an example of error handling in a Flask web API:
from flask import Flask, jsonifyapp = Flask(__name__)
def get_data():
try:
# Attempt to retrieve data
data = retrieve_data()
return jsonify(data)
except Exception as e:
# Handle error and return error response
return jsonify({'error': str(e)}), 500
if __name__ == '__main__':
app.run(debug=True)
In this example, we define a custom error handler to catch and handle exceptions raised during data retrieval.
- Authentication and Authorization: Authentication and authorization are crucial for securing web APIs and controlling access to resources based on user identity and permissions. Flask provides various extensions and middleware for implementing authentication and authorization mechanisms, such as JWT (JSON Web Tokens), OAuth, and session-based authentication. Here’s an example of implementing JWT-based authentication in a Flask web API:
from flask import Flask, jsonify, request
from flask_jwt_extended import JWTManager, jwt_required, create_access_tokenapp = Flask(__name__)
app.config['JWT_SECRET_KEY'] = 'secret'
jwt = JWTManager(app)
def login():
username = request.json.get('username')
password = request.json.get('password')
# Validate credentials
if username == 'admin' and password == 'password':
access_token = create_access_token(identity=username)
return jsonify({'access_token': access_token})
else:
return jsonify({'error': 'Invalid credentials'}), 401
def protected_data():
# Return protected data
return jsonify({'message': 'Protected data'})
if __name__ == '__main__':
app.run(debug=True)
In this example, we use the Flask JWT Extended extension to implement JWT-based authentication and protect a route ‘/api/data’ using the @jwt_required decorator.
- Best Practices for Web API Development in Flask: To develop robust, scalable, and maintainable web APIs in Flask, consider following these best practices:
- Design clear and consistent API endpoints: Use meaningful and consistent naming conventions for API endpoints to make them easy to understand and use.
- Use HTTP status codes appropriately: Use standard HTTP status codes (e.g., 200 for success, 400 for client errors, 500 for server errors) to indicate the outcome of API requests accurately.
- Implement versioning: Consider implementing API versioning to manage changes and updates to API endpoints and maintain backward compatibility with existing clients.
- Validate input data: Validate and sanitize input data from clients to prevent security vulnerabilities and ensure data integrity.
- Handle errors gracefully: Implement robust error handling and provide informative error messages to clients to help them troubleshoot issues effectively.
- Secure sensitive data: Use encryption, hashing, and other security measures to protect sensitive data transmitted over the network and stored on the server.
- Test thoroughly: Write comprehensive unit tests, integration tests, and end-to-end tests to verify the correctness, performance, and reliability of your web APIs.
- Document your APIs: Provide clear and comprehensive documentation for your web APIs, including endpoint descriptions, request parameters, response formats, and error codes, to help developers understand and use your APIs effectively.
- Conclusion: In conclusion, mastering web API development in Flask is essential for building scalable, secure, and maintainable APIs to serve data and interact with clients over the web. By understanding RESTful architecture, leveraging Flask’s features and extensions, implementing authentication and authorization mechanisms, and following best practices, you can develop robust and reliable web APIs that meet the needs of your applications and users. So dive into Flask web API development, practice these techniques, and unlock the full potential of Flask for building powerful and flexible web APIs.