How to Work with JSON in Python
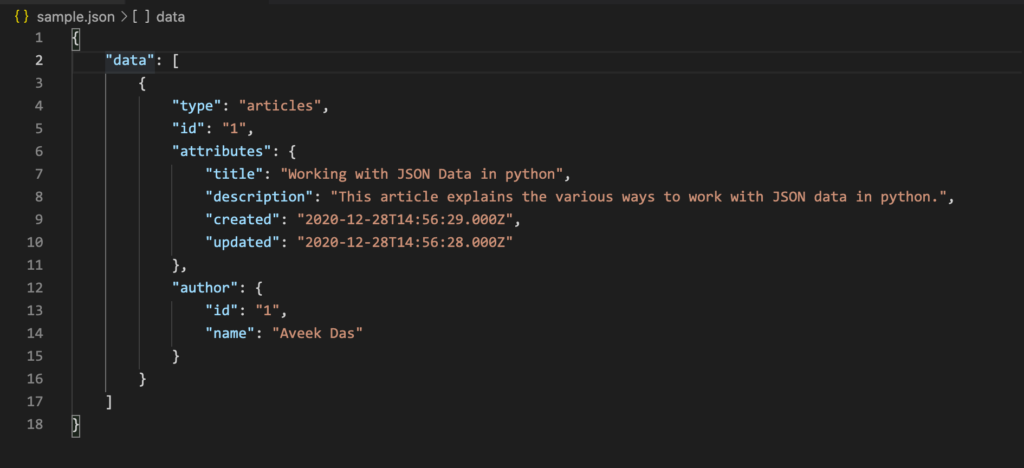
JSON (JavaScript Object Notation) is a lightweight data-interchange format that’s easy for humans to read and write and easy for machines to parse and generate. Python offers extensive support for working with JSON through its json
module. This article will provide a comprehensive guide on how to work with JSON in Python, covering everything from basic concepts to advanced techniques.
Introduction to JSON
JSON is a text format that is language-independent but uses conventions familiar to programmers of the C family of languages, including Python, JavaScript, C++, and many others. JSON data is represented as key-value pairs and supports various data types, including strings, numbers, arrays, booleans, and nulls.
Here’s a simple example of JSON data:
{
"name": "John",
"age": 30,
"is_student": false,
"courses": ["Math", "Science"],
"address": {
"street": "123 Main St",
"city": "Anytown"
}
}
The json
Module in Python
Python’s json
module provides functions for parsing JSON strings and converting Python objects to JSON strings. The primary functions you’ll use are:
json.load()
: Parse JSON data from a file.json.loads()
: Parse JSON data from a string.json.dump()
: Write JSON data to a file.json.dumps()
: Convert Python objects to a JSON string.
Parsing JSON
Parsing JSON from a String
You can parse JSON data from a string using json.loads()
:
import jsonjson_data = '{"name": "John", "age": 30, "is_student": false}'
python_obj = json.loads(json_data)
print(python_obj)
# Output: {'name': 'John', 'age': 30, 'is_student': False}
Parsing JSON from a File
To parse JSON data from a file, use json.load()
:
with open('data.json', 'r') as file:
python_obj = json.load(file)print(python_obj)
# Output: {'name': 'John', 'age': 30, 'is_student': False}
Creating JSON
Creating JSON from a Python Object
To convert a Python object to a JSON string, use json.dumps()
:
python_obj = {
"name": "John",
"age": 30,
"is_student": False,
"courses": ["Math", "Science"],
"address": {
"street": "123 Main St",
"city": "Anytown"
}
}json_data = json.dumps(python_obj)
print(json_data)
# Output: {"name": "John", "age": 30, "is_student": false, "courses": ["Math", "Science"], "address": {"street": "123 Main St", "city": "Anytown"}}
Writing JSON to a File
To write JSON data to a file, use json.dump()
:
with open('data.json', 'w') as file:
json.dump(python_obj, file)
Pretty-Printing JSON
For better readability, you can pretty-print JSON data using the indent
parameter in json.dumps()
or json.dump()
:
pretty_json = json.dumps(python_obj, indent=4)
print(pretty_json)
Output:
{
"name": "John",
"age": 30,
"is_student": false,
"courses": [
"Math",
"Science"
],
"address": {
"street": "123 Main St",
"city": "Anytown"
}
}
Handling Complex Data Types
Custom Serialization
If you need to serialize custom Python objects, you can define a custom serialization function and pass it to json.dumps()
using the default
parameter:
import json
from datetime import datetimeclass User:
def __init__(self, name, birthdate):
self.name = name
self.birthdate = birthdate
def serialize(obj):
if isinstance(obj, datetime):
return obj.isoformat()
elif isinstance(obj, User):
return {'name': obj.name, 'birthdate': obj.birthdate.isoformat()}
raise TypeError(f'Type {type(obj)} not serializable')
user = User('John', datetime(1990, 5, 15))
json_data = json.dumps(user, default=serialize)
print(json_data)
# Output: {"name": "John", "birthdate": "1990-05-15T00:00:00"}
Custom Deserialization
To deserialize JSON data into custom Python objects, use the object_hook
parameter of json.loads()
:
def deserialize(dct):
if 'birthdate' in dct:
dct['birthdate'] = datetime.fromisoformat(dct['birthdate'])
return dctjson_data = '{"name": "John", "birthdate": "1990-05-15T00:00:00"}'
user = json.loads(json_data, object_hook=deserialize)
print(user)
# Output: {'name': 'John', 'birthdate': datetime.datetime(1990, 5, 15, 0, 0)}
Working with JSON in Real-World Scenarios
Example 1: Reading Configuration Files
JSON is often used for configuration files. Here’s how to read and use a JSON configuration file:
import jsonwith open('config.json', 'r') as file:
config = json.load(file)
print(config['database']['host'])
# Output: localhost
Example 2: Sending JSON Data Over HTTP
When working with web APIs, you’ll often need to send and receive JSON data. Here’s an example using the requests
library:
import requests
import jsonurl = 'https://api.example.com/data'
data = {'key': 'value'}
headers = {'Content-Type': 'application/json'}
response = requests.post(url, data=json.dumps(data), headers=headers)
print(response.json())
Example 3: Logging in JSON Format
Logging in JSON format can be useful for structured logging. Here’s an example using the logging
module:
import logging
import jsonclass JsonFormatter(logging.Formatter):
def format(self, record):
log_record = {
'level': record.levelname,
'message': record.msg,
'time': self.formatTime(record, self.datefmt),
}
return json.dumps(log_record)
logger = logging.getLogger()
handler = logging.StreamHandler()
formatter = JsonFormatter()
handler.setFormatter(formatter)
logger.addHandler(handler)
logger.setLevel(logging.INFO)
logger.info('This is a log message')
Output:
{"level": "INFO", "message": "This is a log message", "time": "2024-07-21 12:00:00"}
Error Handling in JSON Operations
Handling errors gracefully is crucial when working with JSON data. Here are some common errors and how to handle them:
JSONDecodeError
This error occurs when JSON data is malformed. Use a try-except block to catch and handle this error:
import jsonjson_data = '{"name": "John", "age": 30, "is_student": false'
try:
python_obj = json.loads(json_data)
except json.JSONDecodeError as e:
print(f'Error decoding JSON: {e}')
# Output: Error decoding JSON: Expecting ',' delimiter: line 1 column 41 (char 40)
TypeError
This error occurs when attempting to serialize an unsupported data type. Define a custom serialization function to handle this error:
import jsonclass CustomType:
pass
try:
json_data = json.dumps(CustomType())
except TypeError as e:
print(f'Error serializing object: {e}')
# Output: Error serializing object: Object of type CustomType is not JSON serializable
JSON Schema Validation
To ensure that JSON data adheres to a specific structure, you can use JSON Schema validation. The jsonschema
library provides support for this:
from jsonschema import validate, ValidationErrorschema = {
"type": "object",
"properties": {
"name": {"type": "string"},
"age": {"type": "number"},
"is_student": {"type": "boolean"}
},
"required": ["name", "age", "is_student"]
}
json_data = {"name": "John", "age": 30, "is_student": False}
try:
validate(instance=json_data, schema=schema)
print('JSON data is valid')
except ValidationError as e:
print(f'JSON data is invalid: {e}')
Tips for Working with JSON
- Keep JSON Data Simple: Avoid deeply nested structures to keep JSON data readable and manageable.
- Use JSONLint: For validating and formatting JSON data, use tools like JSONLint.
- Documentation: Ensure your JSON data is well-documented to help others understand the structure and purpose of the data.
Conclusion
Working with JSON in Python is straightforward thanks to the json
module and its functions. By understanding how to parse, create, and manipulate JSON data, you can handle various tasks such as reading configuration files, sending data over the web, and logging in a structured format. Advanced techniques like custom serialization, error handling, and schema validation further enhance your ability to work with JSON effectively. Whether you’re dealing with small datasets or large-scale applications, mastering JSON in Python is an essential skill for any developer.