How to Use Arrow Functions in JavaScript
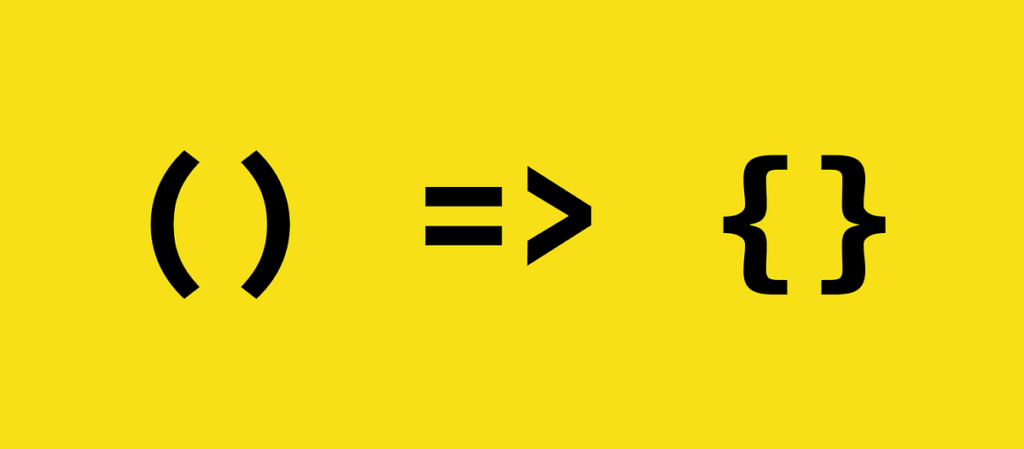
Arrow functions, introduced in ECMAScript 6 (ES6), are a more concise syntax for writing function expressions in JavaScript. They offer a number of benefits, such as shorter syntax, implicit returns, and lexical scoping of the this
keyword. This comprehensive guide will explore everything you need to know about arrow functions, from their basic usage to advanced concepts and best practices.
Table of Contents
- Introduction to Arrow Functions
- Syntax and Basic Usage
- Basic Syntax
- Implicit Return
- Returning Objects
- Lexical Scoping of
this
- Differences Between Regular Functions and Arrow Functions
- Practical Examples
- Arrow Functions and Parameters
- Single Parameter
- Multiple Parameters
- Default Parameters
- Rest Parameters
- Advanced Concepts
- Arrow Functions in Methods
- Arrow Functions in Callbacks
- Arrow Functions in Promises
- Limitations and Caveats
- Performance Considerations
- Best Practices
- When to Use Arrow Functions
- When Not to Use Arrow Functions
- Real-World Examples
- Using Arrow Functions in Array Methods
- Using Arrow Functions with Event Listeners
- Using Arrow Functions in Asynchronous Code
1. Introduction to Arrow Functions
Arrow functions provide a concise way to write function expressions in JavaScript. They are particularly useful for short functions and when you need to maintain the this
context from the surrounding code. Arrow functions do not have their own this
, arguments
, super
, or new.target
bindings, making them unsuitable for certain use cases but highly effective for others.
2. Syntax and Basic Usage
Basic Syntax
The syntax of an arrow function is much shorter than that of a traditional function expression. Here’s a comparison:
Traditional Function Expression:
const add = function(a, b) {
return a + b;
};
Arrow Function:
const add = (a, b) => {
return a + b;
};
Implicit Return
For functions with a single expression, you can omit the braces and the return
keyword. This is known as an implicit return.
const add = (a, b) => a + b;
Returning Objects
When returning an object literal, you need to wrap the object in parentheses to avoid ambiguity with the function body.
const createUser = (name, age) => ({ name, age });
3. Lexical Scoping of this
One of the key differences between arrow functions and traditional functions is how they handle the this
keyword. Arrow functions do not have their own this
context. Instead, they inherit this
from the parent scope at the time they are defined. This is known as lexical scoping of this
.
Differences Between Regular Functions and Arrow Functions
Traditional Function:
function Person() {
this.age = 0; setInterval(function growUp() {
this.age++;
}, 1000);
}
const p = new Person();
console.log(p.age); // NaN, because `this` is not bound correctly
Arrow Function:
function Person() {
this.age = 0; setInterval(() => {
this.age++;
}, 1000);
}
const p = new Person();
console.log(p.age); // Correctly increments age
Practical Examples
Example 1: Event Handlers
When using traditional functions, you often need to use .bind(this)
to maintain the correct this
context.
function Button() {
this.label = 'Click me'; this.handleClick = function() {
console.log(this.label);
};
document.querySelector('button').addEventListener('click', this.handleClick.bind(this));
}
const btn = new Button();
With arrow functions, you don’t need to bind this
.
function Button() {
this.label = 'Click me'; document.querySelector('button').addEventListener('click', () => {
console.log(this.label);
});
}
const btn = new Button();
Example 2: Array Methods
Arrow functions are particularly useful when used with array methods such as map
, filter
, and reduce
.
const numbers = [1, 2, 3, 4, 5];const squares = numbers.map(n => n * n);
console.log(squares); // [1, 4, 9, 16, 25]
4. Arrow Functions and Parameters
Single Parameter
If an arrow function has a single parameter, you can omit the parentheses.
const greet = name => `Hello, ${name}!`;
Multiple Parameters
For multiple parameters, parentheses are required.
const add = (a, b) => a + b;
Default Parameters
Arrow functions can also have default parameters.
const greet = (name = 'Guest') => `Hello, ${name}!`;
Rest Parameters
Rest parameters allow an indefinite number of arguments to be passed to a function as an array.
const sum = (...numbers) => numbers.reduce((total, num) => total + num, 0);
5. Advanced Concepts
Arrow Functions in Methods
While arrow functions are useful in many scenarios, they should not be used as methods of objects because they do not have their own this
context.
const obj = {
value: 42,
logValue: () => {
console.log(this.value); // undefined, because `this` is not bound correctly
}
};obj.logValue();
Arrow Functions in Callbacks
Arrow functions are particularly useful for concise and readable callbacks.
const numbers = [1, 2, 3, 4, 5];const evenNumbers = numbers.filter(n => n % 2 === 0);
console.log(evenNumbers); // [2, 4]
Arrow Functions in Promises
Arrow functions can make promise chains more readable.
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Limitations and Caveats
- No
this
,arguments
,super
, ornew.target
: Arrow functions do not have their own bindings to these keywords. - Not suitable for methods: As discussed earlier, arrow functions should not be used as methods of objects.
- Cannot be used as constructors: Arrow functions cannot be used with the
new
keyword.
6. Performance Considerations
In most cases, the performance difference between arrow functions and traditional functions is negligible. However, it’s essential to understand that arrow functions are not a replacement for all function expressions, and they should be used where appropriate.
7. Best Practices
When to Use Arrow Functions
- For short, concise functions: Arrow functions are ideal for short functions, especially when used as callbacks.
- To maintain
this
context: Use arrow functions to preserve thethis
context from the parent scope. - In array methods: Arrow functions make code more concise and readable when used with array methods like
map
,filter
, andreduce
.
When Not to Use Arrow Functions
- As object methods: Avoid using arrow functions as methods of objects since they do not have their own
this
context. - As constructors: Arrow functions cannot be used as constructors and will throw an error if used with the
new
keyword.
8. Real-World Examples
Using Arrow Functions in Array Methods
Arrow functions make array methods more concise and readable.
Example: Mapping an array
const numbers = [1, 2, 3, 4, 5];const squares = numbers.map(n => n * n);
console.log(squares); // [1, 4, 9, 16, 25]
Example: Filtering an array
const numbers = [1, 2, 3, 4, 5];const evenNumbers = numbers.filter(n => n % 2 === 0);
console.log(evenNumbers); // [2, 4]
Using Arrow Functions with Event Listeners
Arrow functions help maintain the correct this
context when used with event listeners.
function Button() {
this.label = 'Click me'; document.querySelector('button').addEventListener('click', () => {
console.log(this.label);
});
}
const btn = new Button();
Using Arrow Functions in Asynchronous Code
Arrow functions can make asynchronous code, such as promise chains, more readable.
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Conclusion
Arrow functions are a powerful and concise way to write functions in JavaScript. They offer benefits such as shorter syntax, implicit returns, and lexical scoping of this
, making them particularly useful for callbacks and array methods. However, it’s important to understand their limitations and use them appropriately. By following the best practices outlined in this article, you can effectively leverage arrow functions to write clean, maintainable, and efficient JavaScript code.