How to Create and Use Functions in JavaScript
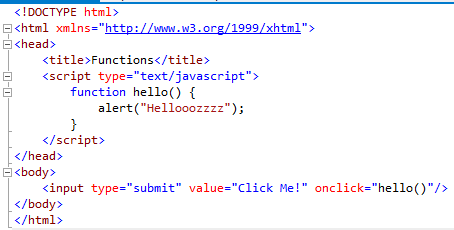
Functions are a fundamental building block in JavaScript and are essential for writing modular, reusable, and maintainable code. This article will cover everything you need to know about creating and using functions in JavaScript, including different types of functions, advanced techniques, and best practices.
Table of Contents
- Introduction to Functions
- Defining Functions
- Function Declarations
- Function Expressions
- Arrow Functions
- Invoking Functions
- Basic Invocation
- Function Parameters and Arguments
- Default Parameters
- Rest Parameters
- Return Values
- Returning Values
- Early Returns
- Scope and Closures
- Function Scope
- Block Scope
- Lexical Scope and Closures
- Advanced Function Concepts
- Immediately Invoked Function Expressions (IIFE)
- Higher-Order Functions
- Callback Functions
- Recursion
- Function Methods and Properties
call
,apply
, andbind
- Function Properties
- Best Practices
- Naming Conventions
- Pure Functions
- Avoiding Side Effects
- Function Documentation
- Real-World Examples
- Utility Functions
- Event Handlers
- Asynchronous Functions
1. Introduction to Functions
Functions are reusable blocks of code that perform a specific task. They can take inputs, process those inputs, and return an output. Functions help to organize code, making it more readable, maintainable, and reusable.
2. Defining Functions
There are several ways to define functions in JavaScript: function declarations, function expressions, and arrow functions.
Function Declarations
A function declaration defines a function with the specified parameters.
function greet(name) {
return `Hello, ${name}!`;
}
Function Expressions
A function expression defines a function as part of a larger expression syntax. They can be named or anonymous.
const greet = function(name) {
return `Hello, ${name}!`;
};
Arrow Functions
Arrow functions provide a shorter syntax for writing functions. They are anonymous and do not have their own this
context.
const greet = (name) => {
return `Hello, ${name}!`;
};// If the function body has only a single statement, you can omit the braces and the return keyword.
const greet = name => `Hello, ${name}!`;
3. Invoking Functions
Basic Invocation
To invoke or call a function, use the function name followed by parentheses.
greet('Alice'); // "Hello, Alice!"
Function Parameters and Arguments
Functions can accept parameters, which are specified when the function is defined, and arguments, which are the actual values passed to the function when it is called.
function add(a, b) {
return a + b;
}add(2, 3); // 5
Default Parameters
Default parameters allow you to specify default values for function parameters.
function greet(name = 'Guest') {
return `Hello, ${name}!`;
}greet(); // "Hello, Guest!"
greet('Alice'); // "Hello, Alice!"
Rest Parameters
Rest parameters allow a function to accept an indefinite number of arguments as an array.
function sum(...numbers) {
return numbers.reduce((total, number) => total + number, 0);
}sum(1, 2, 3); // 6
sum(4, 5, 6, 7, 8); // 30
4. Return Values
Returning Values
Functions can return values using the return
statement.
function multiply(a, b) {
return a * b;
}const result = multiply(2, 3); // 6
Early Returns
You can use early returns to exit a function before it reaches the end.
function divide(a, b) {
if (b === 0) {
return 'Error: Division by zero';
}
return a / b;
}divide(6, 2); // 3
divide(6, 0); // "Error: Division by zero"
5. Scope and Closures
Function Scope
Variables declared inside a function are in the function scope and cannot be accessed outside the function.
function example() {
let message = 'Hello, World!';
console.log(message);
}example(); // "Hello, World!"
console.log(message); // ReferenceError: message is not defined
Block Scope
Variables declared with let
and const
inside a block (e.g., inside a loop or an if
statement) are block-scoped.
if (true) {
let blockMessage = 'Hello from block scope';
console.log(blockMessage); // "Hello from block scope"
}console.log(blockMessage); // ReferenceError: blockMessage is not defined
Lexical Scope and Closures
Closures are functions that have access to the parent scope, even after the parent function has closed.
function outerFunction(outerVariable) {
return function innerFunction(innerVariable) {
console.log('Outer Variable:', outerVariable);
console.log('Inner Variable:', innerVariable);
};
}const newFunction = outerFunction('outside');
newFunction('inside');
// Outer Variable: outside
// Inner Variable: inside
6. Advanced Function Concepts
Immediately Invoked Function Expressions (IIFE)
An IIFE is a function that runs as soon as it is defined.
(function() {
console.log('This function runs immediately');
})();
Higher-Order Functions
Higher-order functions are functions that can take other functions as arguments or return functions as their result.
function higherOrderFunction(callback) {
return callback(5);
}function multiplyByTwo(number) {
return number * 2;
}
const result = higherOrderFunction(multiplyByTwo); // 10
Callback Functions
Callback functions are functions passed into another function as an argument, which is then invoked inside the outer function.
function fetchData(callback) {
// Simulate an API call
setTimeout(() => {
const data = 'Data fetched from API';
callback(data);
}, 1000);
}function handleData(data) {
console.log(data);
}
fetchData(handleData); // "Data fetched from API"
Recursion
Recursion is a technique where a function calls itself.
function factorial(n) {
if (n === 0) {
return 1;
}
return n * factorial(n - 1);
}factorial(5); // 120
7. Function Methods and Properties
call
, apply
, and bind
These methods allow you to set the this
value explicitly.
call
: Invokes the function with a giventhis
value and arguments provided individually.apply
: Invokes the function with a giventhis
value and arguments provided as an array.bind
: Returns a new function, with a giventhis
value and initial arguments.
const person = {
firstName: 'John',
lastName: 'Doe',
fullName: function() {
return `${this.firstName} ${this.lastName}`;
}
};const anotherPerson = {
firstName: 'Jane',
lastName: 'Smith'
};
person.fullName.call(anotherPerson); // "Jane Smith"
person.fullName.apply(anotherPerson); // "Jane Smith"
const fullName = person.fullName.bind(anotherPerson);
fullName(); // "Jane Smith"
Function Properties
Functions in JavaScript are objects and can have properties and methods.
function greet(name) {
return `Hello, ${name}!`;
}greet.language = 'English';
console.log(greet.language); // "English"
8. Best Practices
Naming Conventions
Use clear and descriptive names for functions. A good function name should describe what the function does.
function calculateTotalPrice(price, tax) {
return price + tax;
}
Pure Functions
Pure functions are functions that do not have side effects and always produce the same output for the same input.
function add(a, b) {
return a + b;
}
Avoiding Side Effects
Avoid functions that modify global variables or external states.
let counter = 0;function increment() {
counter += 1;
}
increment(); // counter is now 1
Function Documentation
Use comments and JSDoc to document your functions, especially for complex logic or public APIs.
/**
* Calculates the total price with tax.
*
* @param {number} price - The base price.
* @param {number} tax - The tax amount.
* @returns {number} The total price.
*/
function calculateTotalPrice(price, tax) {
return price + tax;
}
9. Real-World Examples
Utility Functions
Utility functions perform common tasks that can be reused across different parts of an application.
function formatCurrency(amount) {
return `$${amount.toFixed(2)}`;
}console.log(formatCurrency(1234.567)); // "$1234.57"
Event Handlers
Functions that handle events, such as clicks, form submissions, or window resizing.
document.getElementById('myButton').addEventListener('click', function() {
console.log('Button clicked!');
});
Asynchronous Functions
Functions that handle asynchronous operations, such as fetching data from an API.
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error fetching data:', error);
}
}fetchData();
Conclusion
Functions are a core feature of JavaScript that enable developers to write modular, reusable, and maintainable code. By understanding how to create and use functions, manage scope and closures, and apply advanced techniques, you can write more efficient and effective JavaScript programs.
Remember to follow best practices, such as using clear naming conventions, avoiding side effects, and documenting your functions, to ensure your code remains clean and easy to understand. With these skills, you’ll be well-equipped to handle a wide range of programming tasks and challenges in JavaScript.