Mastering XML Data Manipulation in Python: Techniques, Libraries, and Best Practices
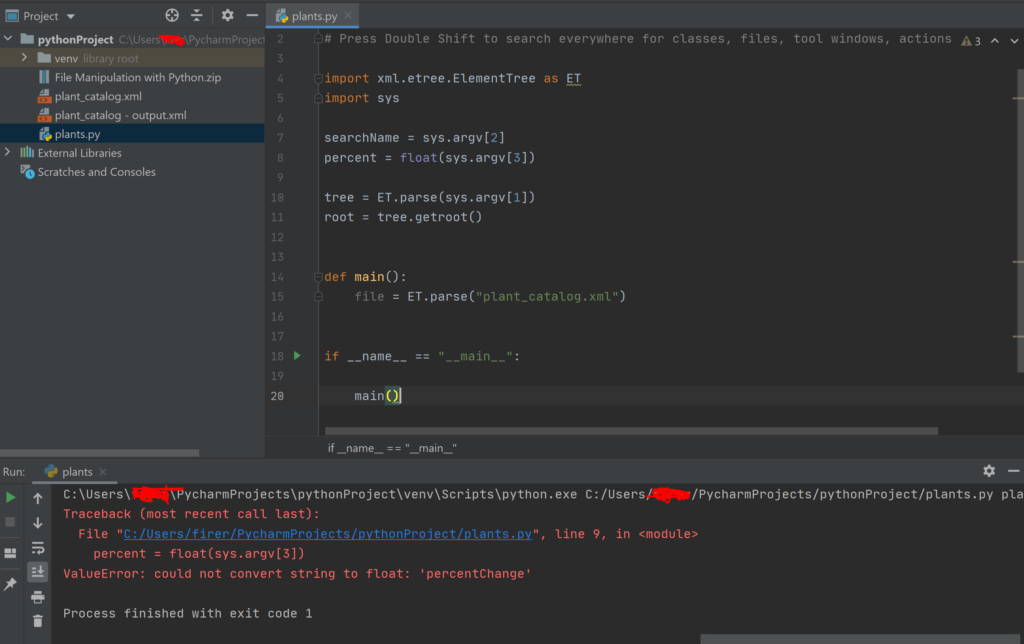
Introduction: XML (eXtensible Markup Language) is a popular format for storing and exchanging structured data, commonly used in web development, data interchange, and configuration files. Python provides a rich set of libraries and tools for manipulating XML data, allowing developers to parse, create, modify, and transform XML documents with ease. By mastering XML data manipulation in Python, you can effectively work with XML-based data sources, integrate XML data into your applications, and automate XML processing tasks. In this comprehensive guide, we’ll explore everything you need to know about manipulating XML data in Python, from basic parsing to advanced transformation techniques and best practices.
- Understanding XML Basics: Before diving into XML data manipulation in Python, it’s essential to understand some fundamental concepts:
- XML Structure: XML documents consist of nested elements, each enclosed in start and end tags. Elements can contain text, attributes, and child elements.
- XML Namespace: XML namespaces provide a way to avoid element name conflicts by associating element names with unique identifiers.
- XML Schema: XML Schema Definition (XSD) is a standard for describing the structure and constraints of XML documents.
- Parsing XML Data in Python: Python provides several libraries for parsing XML data, including xml.etree.ElementTree and lxml. Here’s how to parse XML data using xml.etree.ElementTree:
import xml.etree.ElementTree as ET# Parse XML from a file
tree = ET.parse('data.xml')
# Get the root element
root = tree.getroot()
# Access elements and attributes
for child in root:
print(child.tag, child.attrib)
- Modifying XML Data in Python: Once you’ve parsed an XML document, you can modify its structure, content, and attributes programmatically. Here’s how to modify XML data in Python using xml.etree.ElementTree:
# Modify element text
for child in root:
child.text = 'modified'# Add new elements
new_element = ET.Element('new_element')
new_element.text = 'new text'
root.append(new_element)
# Save modified XML to a new file
tree.write('modified_data.xml')
- Using XPath for XML Data Manipulation: XPath is a powerful query language for selecting nodes in XML documents, allowing you to perform complex searches and manipulations. Python’s lxml library provides XPath support for querying and modifying XML data. Here’s how to use XPath with lxml in Python:
from lxml import etree# Parse XML from a file
tree = etree.parse('data.xml')
# Select nodes using XPath
nodes = tree.xpath('//element[@attribute="value"]')
# Modify selected nodes
for node in nodes:
node.text = 'modified'
# Save modified XML to a new file
tree.write('modified_data.xml')
- Transforming XML Data with XSLT: XSLT (eXtensible Stylesheet Language Transformations) is a powerful language for transforming XML documents into different formats. Python’s lxml library provides XSLT support for applying transformations to XML data. Here’s how to use XSLT with lxml in Python:
# Load XSLT stylesheet from a file
xslt = etree.parse('stylesheet.xsl')# Create XSLT transformer
transform = etree.XSLT(xslt)
# Apply transformation to XML data
result = transform(tree)
# Save transformed XML to a new file
result.write('transformed_data.xml')
- Validating XML Data with XML Schema: XML Schema Definition (XSD) allows you to define the structure and constraints of XML documents, providing a way to validate XML data against a schema. Python’s lxml library provides support for validating XML data using XML Schema. Here’s how to validate XML data against an XSD schema in Python:
# Load XML Schema from a file
schema = etree.XMLSchema(etree.parse('schema.xsd'))# Validate XML data against the schema
valid = schema.validate(tree)
if valid:
print('XML data is valid')
else:
print('XML data is not valid')
- Best Practices for Manipulating XML Data in Python: To effectively manipulate XML data in Python and write clean, maintainable code, consider following these best practices:
- Use appropriate libraries: Choose the right XML parsing and manipulation library for your use case, such as xml.etree.ElementTree for simple tasks or lxml for more complex scenarios.
- Validate XML data: Validate XML data against an XML Schema to ensure its correctness and compliance with predefined rules and constraints.
- Modularize code: Break down XML manipulation tasks into smaller, modular functions or methods to improve code readability, reusability, and maintainability.
- Handle errors gracefully: Use error handling mechanisms, such as try-except blocks, to handle parsing errors, validation failures, and other exceptional conditions gracefully.
- Test thoroughly: Write unit tests to verify the correctness of XML manipulation code and cover different scenarios, edge cases, and error conditions.
- Document code and schemas: Document XML manipulation code and XML Schema definitions using comments, docstrings, and annotations to make them understandable and maintainable.
- Conclusion: In conclusion, mastering XML data manipulation in Python is essential for working with XML-based data sources, integrating XML data into your applications, and automating XML processing tasks. By understanding XML basics, parsing XML data, modifying XML structures, applying XPath queries, transforming XML with XSLT, validating XML against schemas, and following best practices, you can effectively manipulate XML data in Python and write clean, maintainable code. So dive into XML data manipulation, practice these techniques, and unlock the full potential of Python for working with XML-based data.