Mastering Dictionary Manipulation in Python: Techniques, Methods, and Best Practices
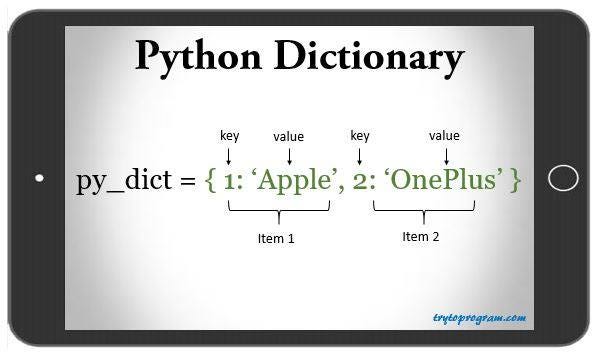
Introduction: Dictionaries are a versatile and powerful data structure in Python, allowing you to store and manipulate key-value pairs efficiently. They provide a flexible way to represent data and are widely used in various programming tasks, such as data processing, web development, and algorithm design. By mastering dictionary manipulation in Python, you can perform a wide range of operations, including adding, accessing, modifying, and iterating over dictionary elements. In this comprehensive guide, we’ll explore everything you need to know about working with dictionaries in Python, from basic concepts to advanced techniques and best practices.
- Understanding Dictionary Basics: A dictionary in Python is an unordered collection of key-value pairs, where each key is unique and maps to a corresponding value. Dictionaries are mutable, meaning you can modify their contents after creation. Here’s a basic example of creating and accessing dictionary elements in Python:
# Create a dictionary
person = {'name': 'John', 'age': 30, 'city': 'New York'}# Access dictionary elements
print(person['name']) # Output: John
print(person['age']) # Output: 30
print(person['city']) # Output: New York
- Dictionary Methods and Operations: Python provides a rich set of methods and operations for working with dictionaries. Here are some common operations you can perform with dictionaries:
- Adding and Updating Elements:
# Add a new key-value pair
person['email'] = 'john@example.com'# Update an existing value
person['age'] = 31
- Removing Elements:
# Remove a key-value pair
del person['city']# Remove all elements
person.clear()
- Checking Membership:
# Check if a key exists
if 'age' in person:
print('Age:', person['age'])
- Iterating Over Elements:
# Iterate over keys
for key in person:
print(key, person[key])# Iterate over key-value pairs
for key, value in person.items():
print(key, value)
- Dictionary Comprehensions: Similar to list comprehensions, Python supports dictionary comprehensions, allowing you to create dictionaries using a concise syntax. Here’s an example of creating a dictionary using a comprehension:
# Create a dictionary of squares
squares = {x: x**2 for x in range(1, 6)}
print(squares) # Output: {1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
- Handling Missing Keys: When accessing dictionary elements, it’s common to encounter situations where the key may not exist. Python provides several methods for handling missing keys, such as using the get method with a default value or using the setdefault method to set a default value if the key is missing:
# Using get method with default value
age = person.get('age', 0)# Using setdefault method
person.setdefault('email', 'unknown@example.com')
- Nested Dictionaries: Dictionaries in Python can contain other dictionaries as values, allowing you to represent hierarchical or nested data structures. Here’s an example of a nested dictionary representing employee information:
employees = {
'john': {'age': 30, 'department': 'Engineering'},
'alice': {'age': 25, 'department': 'Marketing'}
}
- Best Practices for Working with Dictionaries: To write clean, efficient, and maintainable code when working with dictionaries in Python, consider following these best practices:
- Choose meaningful keys: Use descriptive and meaningful keys to represent dictionary elements, making it easier to understand and maintain your code.
- Use dictionary methods: Take advantage of built-in dictionary methods and operations, such as get, items, keys, and values, to perform common tasks efficiently.
- Handle missing keys gracefully: Use appropriate methods, such as get or setdefault, to handle missing keys and avoid KeyError exceptions.
- Prefer dictionary comprehensions: When creating dictionaries from iterable data, prefer dictionary comprehensions over manual iteration for cleaner and more concise code.
- Document your dictionaries: Add comments or docstrings to document the purpose, structure, and usage of dictionaries in your code for clarity and maintainability.
- Test your dictionary manipulation code: Write unit tests to verify the correctness and performance of your dictionary manipulation code, covering different scenarios and edge cases.
- Conclusion: In conclusion, mastering dictionary manipulation in Python is essential for working with key-value data efficiently and effectively. By understanding dictionary basics, using built-in methods and operations, leveraging dictionary comprehensions, handling missing keys gracefully, and following best practices, you can write clean, maintainable, and expressive code that makes full use of Python’s powerful dictionary data structure. So dive into dictionary manipulation, practice these techniques, and unlock the full potential of dictionaries in your Python projects.